Digital Signing
One of the most important concepts in the Integra ecosystem is the concept of digital signing. In this guide, we will provide a detailed overview of how digital signing a hash works and is important in the Integra ecosystem. In addition, code examples, developer notes, and how to implement digital signing of hashes will be explained.
Overview
With the decentralized nature of blockchain and the lack of a central repository, or database, of users it is very difficult to determine who wrote a hash and if the hash was written by a malicious user to show that the modified document is the original. This is where digital signing, or creating a digital signature of the hash, factors in to address the issues of tampering and impersonation using public-key cryptography. While you cannot prevent the data from being altered by someone in the network, the receiving node must be able to detect whether data has been altered and, if so, not pass the corrupted data to the application. A Message digest or one-way hash is used for this purpose. In short, a message digest is a fingerprint of the data. If the data changes, the fingerprint (message digest or hash) changes in ways you cannot predict.
Digital Signing does not encrypt the data but rather it creates a one-way hash of the data and then the private key is used to encrypt the hash. The content of the hashed data cannot be determined from the hash. This is why it is called one-way. This encrypted hash, along with the hashing algorithm and other information, is known as a digital signature.
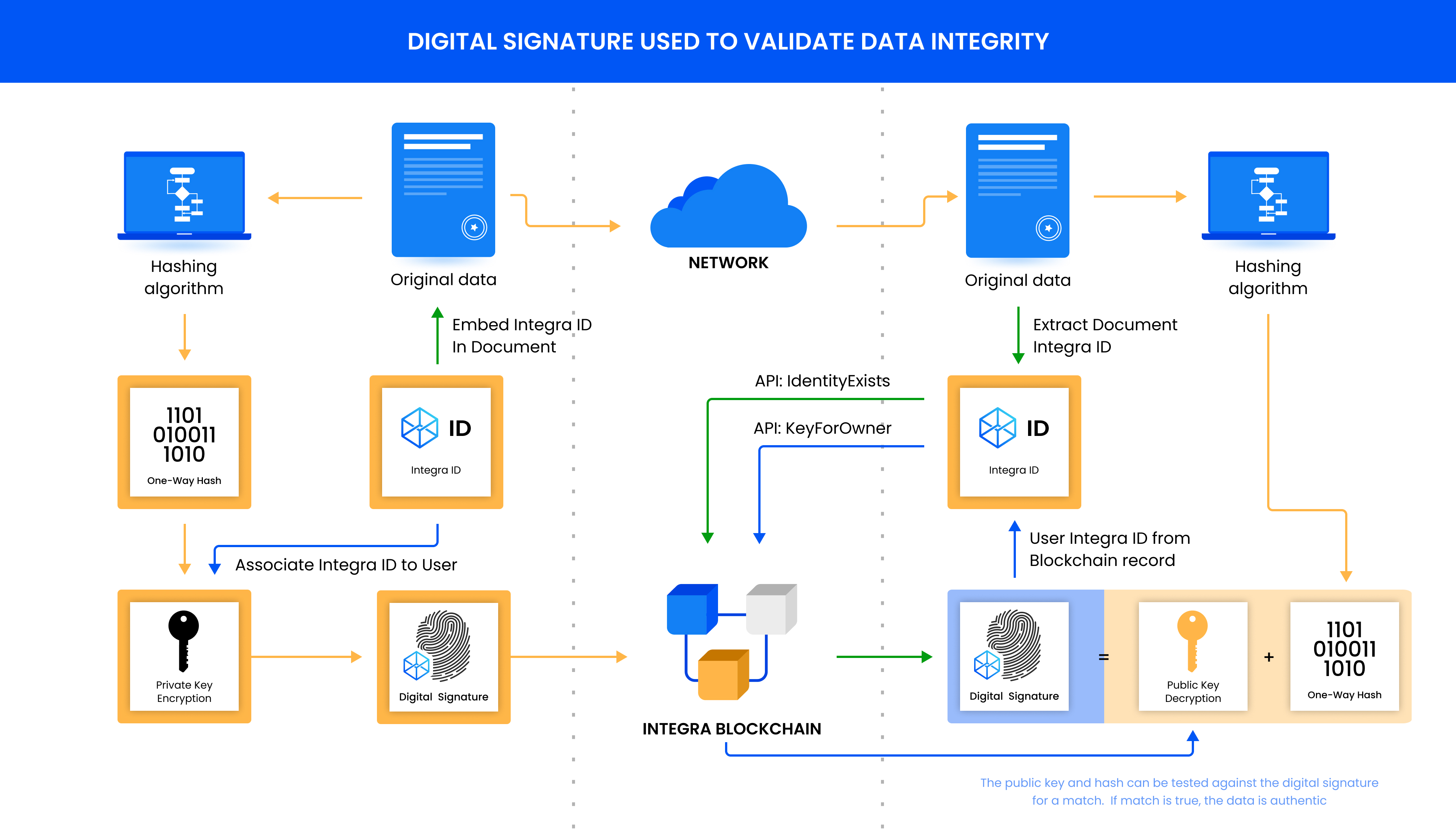
This is how the process of digital signing of a hash works in the Integra Ecosystem. Note that there are two IntegraIds, one for the document and one for the user. The signed value can't be decrypted but can be tested against for a match of the public key and hash.
The original document, with the integraId embedded, is sent to the recipient. Using the one-way hash to validate the integrity of the data or document, the recipient uses the Integra blockchain to look up the sender's public key by using the integraId that is embedded in the document and also registered in the record on the blockchain. Using the hashing algorithm included in the digital signature, the same one-way hash of the document is generated by the recipient. Using the newly created hash along with the public key stored on Integra, the digital signature can be compared to ensure there is a match and that the data has not changed since it was signed. If there is not a match, that means the data has either been tampered with or the private key that created the digital signature does not match the public key stored on the Integra blockchain. Either way, the document is not authentic and or has been tampered with and should not be trusted.
Coding Examples and Understanding
Now that a basic understanding of why digitally signing a hash is important, we look at what goes into this process from a coding point of view. Before diving into code, please remember that the private key is stored by the owner in a secure manner while the public key is stored on the Integra blockchain and can be accessed using KeyForOwner API call by simply providing the integraId included in the document. The first component of the process is creating the hash of the document and then using the private key to one-way encrypt the hash. Below is the Node.js code for digitally signing a hash of a document.
let signedHash;
if (key_data) {
const { pass_phrase: password, private_key: encryptedPrivateKey } = JSON.parse(key_data);
const decryptedPrivateKey = cryptoJs.AES.decrypt(encryptedPrivateKey, password).toString(cryptoJs.enc.Utf8);
const fileData = await readFileAsync(`modified/${fileName}`);
const hash = crypto.createHash('sha256').update(fileData).digest('hex');
signedHash = encryptStringWithRsaPrivateKey(hash, decryptedPrivateKey);
}
const encryptStringWithRsaPrivateKey = (toEncrypt, privateKey) => {
const sign = crypto.createSign('SHA256');
sign.update(toEncrypt);
sign.end();
const signature = sign.sign(privateKey);
return signature.toString('base64');
};
Now that we have generated all of the components for a digital signature, the next step will be to write it to the blockchain using RegisterIdentity.
Ok so now we have demonstrated how to create a hash from a document, digitally sign the hash and register it to the blockchain. So next up is how to verify that the digitally signed hash of the document is authentic and unaltered. In order to do this the first step is always to get the current hash of the document, also known as the current digital fingerprint of the document. The next step in the process is to get the public key associated with the IntegraId that has digitally signed the document. The IntegraId will be in the record for the hash of the document. To get the transaction containing the registered document, use the ValueExists API where the value is the hash of the document. To get the public key use the KeyForOwner API call. Once the hash and public key have been gathered, the code below will check to see if the hash and public key match what was generated by the private key.
Digital Signing of the Hash Information
The digital signing of the hash is not the same as encryption/decryption. Since the digital signature was created with the private key, there is no way to "decrypt" it using the public key. There is however a way to "test" the value of the hash with the public key to see if it does indeed match the digital signature that was generated, this being the proof that the hashes do indeed match.
//calculate the current hash of the file
const fileData = await readFileAsync(`modified/${fileName}`);
const hash = crypto.createHash('sha256').update(fileData).digest('hex');
//check to see if the hash and the public key match the digital signature
const verify = crypto.createVerify('SHA256');
verify.write(hash);
verify.end();
const result = verify.verify(pubKey, Buffer.from(encryptedString, 'base64'));
Updated about 2 years ago